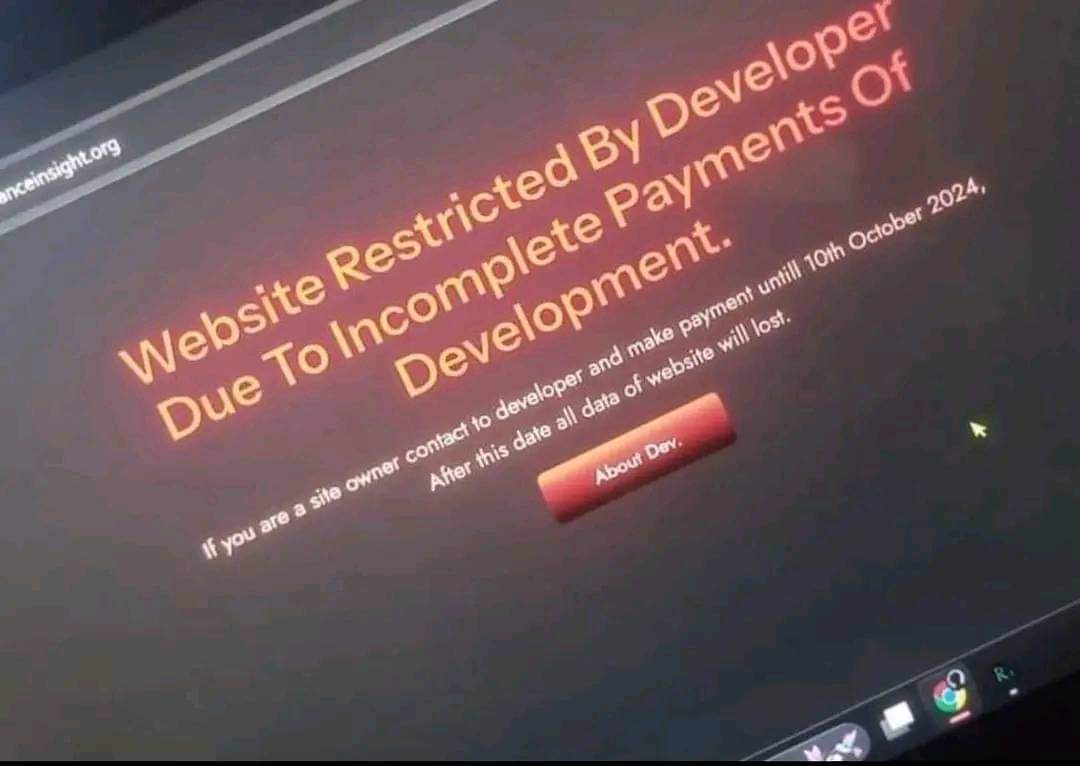
Steps for Implementing Date-Based Restriction in Laravel
1. Create a Restriction View
First, create the restriction page (restriction.blade.php
) in the resources/views
folder.
File: resources/views/restriction.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Restricted</title>
<link rel="stylesheet" href="{{ asset('css/restriction.css') }}">
</head>
<body>
<div class="container">
<h1>Website Restricted By Developer</h1>
<p>Due To Incomplete Payments Of Development.</p>
<p>If you are a site owner, contact the developer and make payment until <strong>10th October 2024</strong>.</p>
<p>After this date, all data of the website will be lost.</p>
<button onclick="alert('Contact Developer')">About Dev.</button>
</div>
</body>
</html>
2. Add Restriction Styles
Add the restriction.css
file to the public/css
directory.
File: public/css/restriction.css
body {
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #1a1a1a;
color: #f2f2f2;
font-family: Arial, sans-serif;
}
.container {
text-align: center;
border: 2px solid #f00;
padding: 20px;
background: #2e2e2e;
border-radius: 10px;
box-shadow: 0 0 15px #f00;
}
h1 {
color: #ff3b3b;
font-size: 2rem;
margin-bottom: 10px;
}
p {
font-size: 1rem;
margin: 10px 0;
}
button {
background-color: #ff3b3b;
color: #fff;
border: none;
padding: 10px 20px;
font-size: 1rem;
cursor: pointer;
border-radius: 5px;
}
button:hover {
background-color: #d32f2f;
}
3. Create Middleware for Restriction
Create a middleware to check the current date and restrict access after the specified date
Run the following command in your terminal to create middleware:
php artisan make:middleware RestrictionMiddleware
Update the middleware logic in app/Http/Middleware/RestrictionMiddleware.php
:
File: app/Http/Middleware/RestrictionMiddleware.php
<?php
namespace App\Http\Middleware;
use Closure;
use Illuminate\Http\Request;
class RestrictionMiddleware
{
public function handle(Request $request, Closure $next)
{
// Set the restriction date
$restrictionDate = now()->create('2024-10-10 23:59:59');
// Check current date and redirect if past the restriction date
if (now()->greaterThan($restrictionDate)) {
return response()->view('restriction');
}
return $next($request);
}
}
4. Register Middleware
Register the middleware in app/Http/Kernel.php
:
File: app/Http/Kernel.php
protected $middlewareGroups = [
'web' => [
// Other middleware...
\App\Http\Middleware\RestrictionMiddleware::class,
],
];
5. Test the Implementation
- After the restriction date (
10th October 2024
), any request to your Laravel website will show the restriction page. - Before the date, the site will function normally.
Optional Customization
- If you want to apply the restriction only to specific routes, use route-specific middleware instead of adding it to the
web
middleware group. - To customize the restriction message dynamically, pass data to the
restriction.blade.php
view.