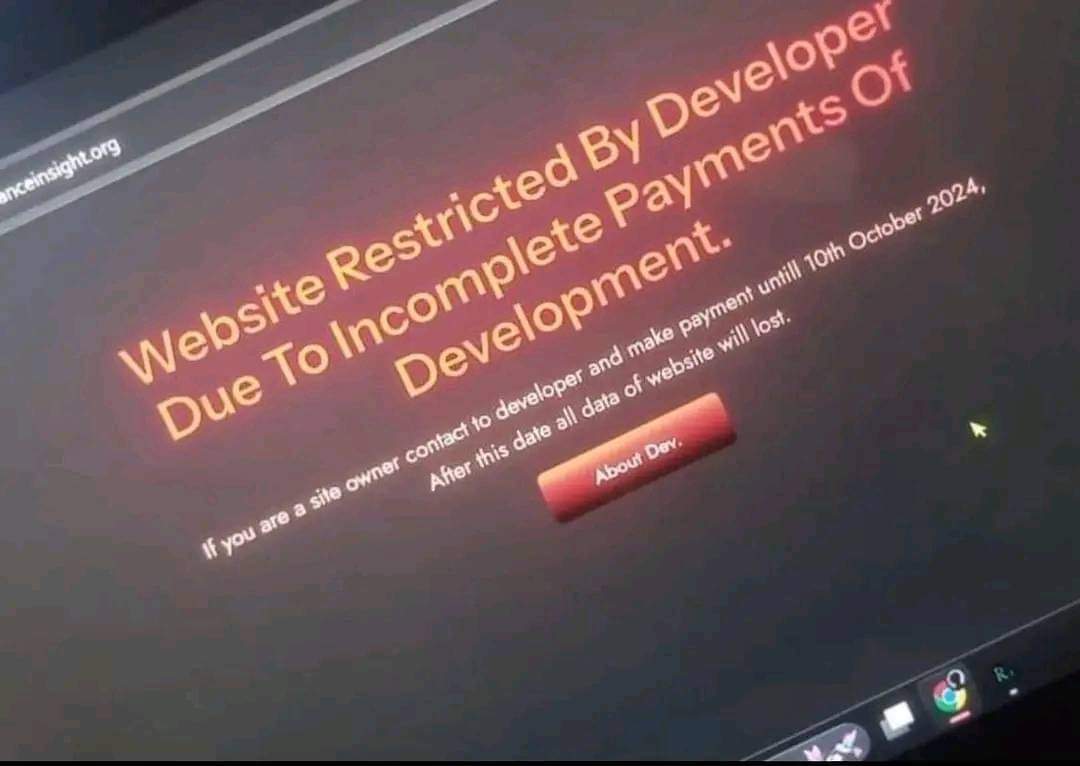
Purpose
To automatically restrict access to a website after a specific date by showing a restriction page. The restriction is implemented using JavaScript to check the date and redirect users.
Steps
1. Main Page (index.html
)
This is the main page of your website. Before the specified restriction date, it will function normally. After the restriction date, it will redirect the user to the restriction page.
File: index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Website</title>
<script>
// Define the restriction date
const restrictionDate = new Date('2024-10-10T23:59:59');
// Check the current date and redirect if past the restriction date
window.onload = function () {
const currentDate = new Date();
if (currentDate > restrictionDate) {
// Redirect to the restriction page
window.location.href = "restriction.html";
}
};
</script>
</head>
<body>
<h1>Welcome to Our Website</h1>
<p>This is the regular content of the website.</p>
</body>
</html>
2. Restriction Page (restriction.html
)
This page will be displayed after the specified restriction date.
File: restriction.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Access Restricted</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="container">
<h1>Access Restricted</h1>
<p>The website is restricted due to incomplete payments for development.</p>
<p>Please contact the developer to resolve this issue before <strong>10th October 2024</strong>.</p>
<p>After this date, all data associated with the website will be lost.</p>
<button onclick="alert('Contact Developer: example@example.com')">Contact Developer</button>
</div>
</body>
</html>
3. CSS File for Styling (styles.css
)
This CSS file styles the restriction page.
File: styles.css
body {
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #1a1a1a;
color: #f2f2f2;
font-family: Arial, sans-serif;
}
.container {
text-align: center;
border: 2px solid #f00;
padding: 20px;
background: #2e2e2e;
border-radius: 10px;
box-shadow: 0 0 15px #f00;
}
h1 {
color: #ff3b3b;
font-size: 2rem;
margin-bottom: 10px;
}
p {
font-size: 1rem;
margin: 10px 0;
}
button {
background-color: #ff3b3b;
color: #fff;
border: none;
padding: 10px 20px;
font-size: 1rem;
cursor: pointer;
border-radius: 5px;
}
button:hover {
background-color: #d32f2f;
}
How It Works
-
Date Check on Page Load:
- The JavaScript in
index.html
checks the current date against the restriction date (2024-10-10
in this case).
- The JavaScript in
-
Redirection Logic:
- If the current date is after the restriction date, the user is automatically redirected to the
restriction.html
page.
- If the current date is after the restriction date, the user is automatically redirected to the
-
Restriction Page:
- The restriction page informs the user why access is restricted and provides contact details for the developer.
Deployment Instructions
- Upload all three files (
index.html
,restriction.html
, andstyles.css
) to your website's directory (e.g.,public_html/
). - Test the functionality by changing the restriction date to a past date and ensuring the redirection works as expected.
- Once verified, set the final restriction date (
2024-10-10
or any desired date).